Do you want to learn how to find web elements from any website in VBA using Selenium? This blog will surely help you to learn this. So, let’s get started.
Table of Contents
- Introduction
- Methods to Locate Web Elements
- Various Methods to Interact with Elements
- Best Practices
- Conclusion
Introduction
In the world of web automation, Selenium has become an essential tool for testers and developers alike. It allows for automated testing and interaction with web applications, making it a powerful asset in the realm of quality assurance and web development.
When working with Selenium in VBA (Visual Basic for Applications), one of the key concepts to understand is web element locators. These locators serve as the bridge between your VBA code and the elements on a web page that you want to interact with.
To practice and run the below code, you need to install Selenium Type Library in VBA to run the below examples. To do so, follow the blog How to Install Selenium Webdriver for VBA in Windows.
After you successfully installed Selenium and ChromeDriver for VBA, use the below code to create a new Chrome webdriver object and open https://www.vpktechnologies.com/ website.
Sub OpenBrowser() Dim driver As New WebDriver driver.Start "chrome" driver. Get " https://www.vpktechnologies.com/" End Sub |
Methods to Locate Web Elements
Web element locators are essentially pointers or identifiers that help Selenium locate and interact with specific elements on a web page. These elements could be buttons, input fields, links, checkboxes, or any other HTML element. Since web pages are structured using HTML, each element is assigned various attributes that can be used to uniquely identify them.
There are several types of locators you can use to target elements:
1. ID Locator
An HTML element can have an id attribute that is unique on the page. This makes it a highly reliable locator. In VBA, you can use the FindElementById() method to locate elements by their id.
Example: We want to locate an element that has an id with the attribute name main as shown in the below image.
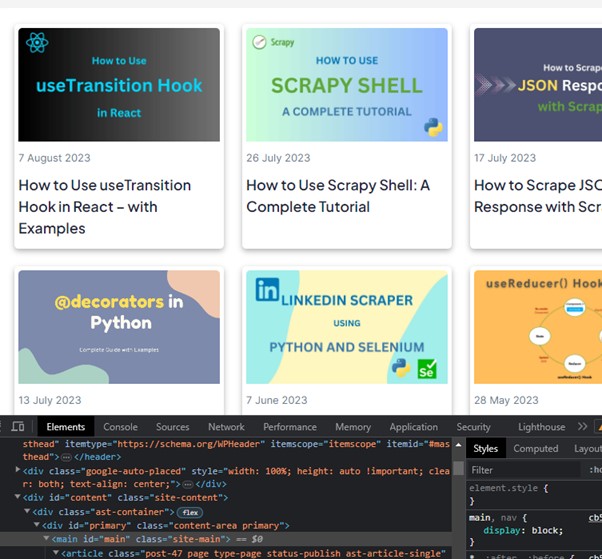
The VBA code to extract inner HTML inside this element is:
Dim allHtml As String allHtml = driver.FindElementById( "main" ).Attribute( "innerHTML" ) |
2. Name Locator
If elements have a name attribute, you can use this attribute to locate them. In VBA, you would use FindElementByName() method.
'Find element by name and add some text driver.FindElementByName( "name" ).SendKeys "myusername" |
For finding more than one element using the Name attribute, use FindElementsByName() method.
driver.FindElementsByName( "name" ) |
3. Class Name Locator
Elements with the class attribute can be located using this locator. The FindElementByClassName() can be used to locate elements using this method.
Dim blogTitle As String blogTitle = driver.FindElementByClass( "wp-block-post-title" ).Attribute( "innerText" ) |
If you want to get more than one element with the same class name, you can use FindElementsByClassName() method as shown in below code:
driver.FindElementsByClass( "wp-block-post-title" ) |
4. Tag Name Locator
This locator finds elements by their HTML tag, such as div, input, a, etc. In VBA, you can use FindElementByTagName() method to locate elements.
Dim blogTitle As String blogTitle = driver.FindElementByTag( "h5" ).Attribute( "innerText" ) |
If you want to get more than one element with the same tag name, you can use FindElementsByTagName() method as shown below code:
driver.FindElementsByTag( "h5" ) |
5. XPath Locator
XPath (XML Path Language) is a powerful language used to navigate XML documents. In Selenium, you can use XPath expressions to locate elements based on their attributes and relationships within the HTML structure. Use FindElementByXPath() method to locate the element.
Dim blogTitle As String blogTitle = driver.FindElementByXPath( "//h5[contains(@class, 'wp-block-post-title')]" ).Attribute( "innerText" ) |
If you want to get more than one element with the same XPath name, you can use FindElementsByXPath() method as shown in below code:
driver.FindElementsByXPath( "//h5[contains(@class, 'wp-block-post-title')]" ) |
6. CSS Selector Locator
Similar to XPath, CSS selectors are used to target elements based on their attributes and relationships, but using CSS syntax. Use FindElementByCss() method to find the element.
Dim blogTitle As String blogTitle = driver.FindElementByCss( "h5[class*='wp-block-post-title']" ).Attribute( "innerText" ) |
If you want to get more than one element with the same CSS name, you can use FindElementsByCss() method as shown below code:
driver.FindElementsByCss( "h5[class*='wp-block-post-title']" ) |
Various Methods to Interact with Elements
When using Selenium with VBA to interact with web elements, you can utilize a variety of methods to perform actions like clicking buttons, entering text, retrieving element attributes, and more. Here’s a list of commonly used methods and properties for element interaction:
1. Click
This method simulates a click action on the element, which is commonly used for buttons, links, and other clickable elements.
driver.FindElementsByTag( "a" ).Click |
2. SendKeys
Use this method to input text into input fields, text areas, and other editable elements.
driver.FindElementByName( "name" ).SendKeys "myusername" |
3. Clear
Clears the content of an input field or text area.
driver.FindElementByName( "name" ).Clear |
4. Submit
If you save the form element in formElement object then you can perform submit method to submit a form element.
formElement.Submit |
5. Attribute
Retrieves the value of a specified attribute of the element. You can get inner text, HTML, or href attribute. As well as you can also get custom attributes like data-id from a given element.
blogTitle = driver.FindElementByCss( "h5" ).Attribute( "innerText" ) blogHTML = driver.FindElementByCss( "h5" ).Attribute( "innerHTML" ) blogURL = driver.FindElementByCss( "h5" ).Attribute( "href" ) |
6. Text
Retrieves the visible text content of an element.
blogTitle = driver.FindElementByCss( "h5" ).Text |
Best Practices
- Use the most reliable locator available. IDs are usually the best choice when available, followed by other locators in order of specificity and uniqueness.
- Avoid using XPath and CSS selectors that are too brittle and can break easily with minor changes to the page’s structure.
- Regularly check and update your locators if the web page’s structure changes.
Conclusion
Selenium web element locators are the foundation of web automation in VBA. By understanding and mastering these locators, you’ll be able to create robust and reliable automated scripts that interact seamlessly with web applications. Whether you’re a QA engineer or a developer, leveraging Selenium’s power in conjunction with VBA can significantly streamline your testing and development processes.
Related: How to Search and Locate Elements in Selenium Python