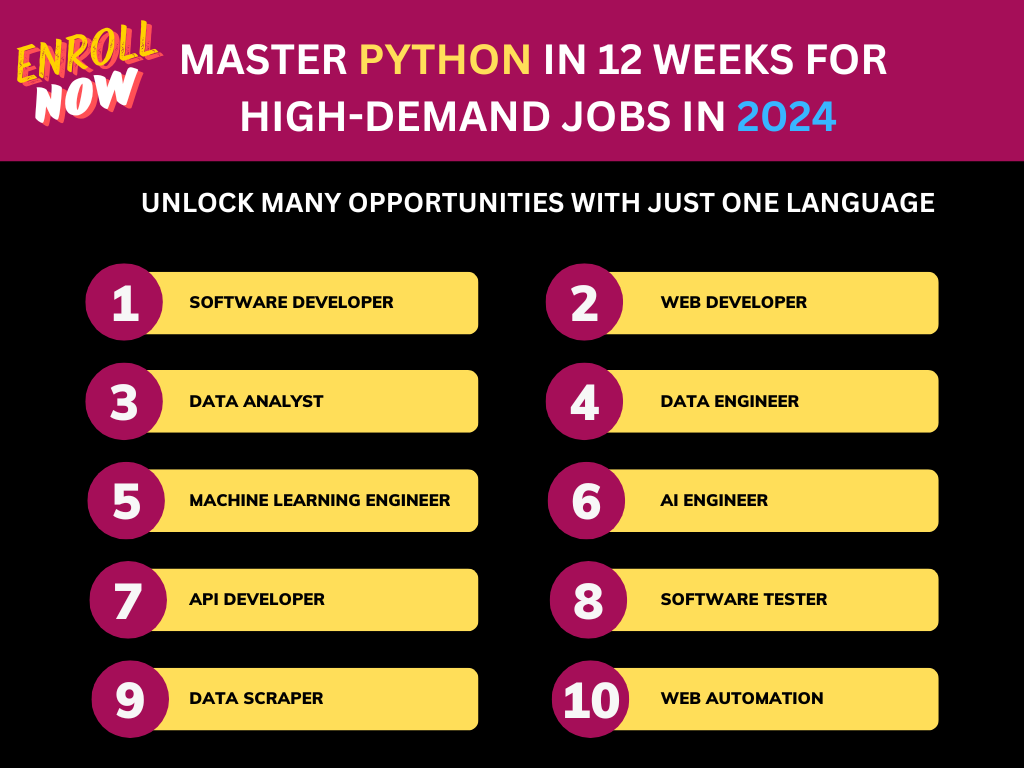
Advanced Python Developer + Internship
Python is a high-level, interpreted programming language known for its simplicity and readability. It’s versatile and widely used across various domains including web development, data science, machine learning, artificial intelligence, automation, and more.
Python emphasizes code readability and encourages a clean and concise coding style, which makes it accessible for beginners and efficient for experienced developers. It offers extensive libraries and frameworks that facilitate rapid development and prototyping.
Syllabus
Introduction to Python
- Overview of Python
- Installing Python
- Setting up the development environment
Python Basics
- Data types (integers, floats, strings, lists, tuples, dictionaries)
- Variables and assignments
- Basic operations (arithmetic, string manipulation)
- Comments and basic syntax
Control Flow
- Conditional statements (if, elif, else)
- Loops (for loops, while loops)
- Control flow keywords (break, continue, pass)
Functions and Modules
- Defining functions
- Function arguments and return values
- Scope and namespaces
- Importing modules
Data Structures
- Lists: Methods and operations
- Tuples: Immutable sequences
- Dictionaries: Key-value pairs
- Sets: Unordered collections of unique elements
File Handling
- Reading from and writing to files
- Working with different file formats (CSV, JSON, etc.)
Exception Handling
- Handling errors and exceptions using try-except blocks
- Handling multiple exceptions
- Using finally block
Object-Oriented Programming (OOP)
- Introduction to OOP concepts (classes, objects, inheritance, polymorphism)
- Defining classes and objects
- Class constructors, methods, and attributes
- Encapsulation, inheritance, and polymorphism
Advanced-Data Structures
- Unpacking in Python
- List comprehensions
- Dictionary comprehensions
- Generators and generator expressions
- Iterators and iterables
- Decorators
Functional Programming
- Lambda functions
- Map, filter, and reduce functions
Regular Expressions
- Introduction to regular expressions
- Using re module for pattern matching
Concurrency and Parallelism
- Threading vs. multiprocessing
- Python’s threading and multiprocessing modules
- Asynchronous programming with async/await
Database Interaction
- Introduction to databases
- Using SQLite with Python
- Using PostgreSQL with Python
Web Development with Python
- Introduction to web frameworks (Django, Flask)
- Creating web applications with Flask
- Handling requests and responses
- Templating and routing
- Creating web applications with Django
Web Scraping
- Overview of Web Scraping
- Web scraping frameworks (requests, Selenium, Beautiful Soup, Scrapy)
- GET, POST with requests framework
- Scraping with Selenium and Beautiful Soup
- Scraping with Scrapy
Version Control
- Introduction to Version Control and Git Basics
- Working with branches
- Collaborating with Git
Container Applications
- Introduction to Docker and Containerization
- Working with Docker Images
Cloud (AWS)
- Introduction to Cloud
- Introduction to AWS
Project Management
- Introduction SDLC
- Introduction to Agile Project Management
- Introduction to JIRA
- SOLID Principles
Fees: ₹10,999
Installment: ₹5,999 + ₹5,000
Duration: 4 Months
Contact us: (+91) 957-900-8270 contact@vpktechnologies.com
Course Pricing
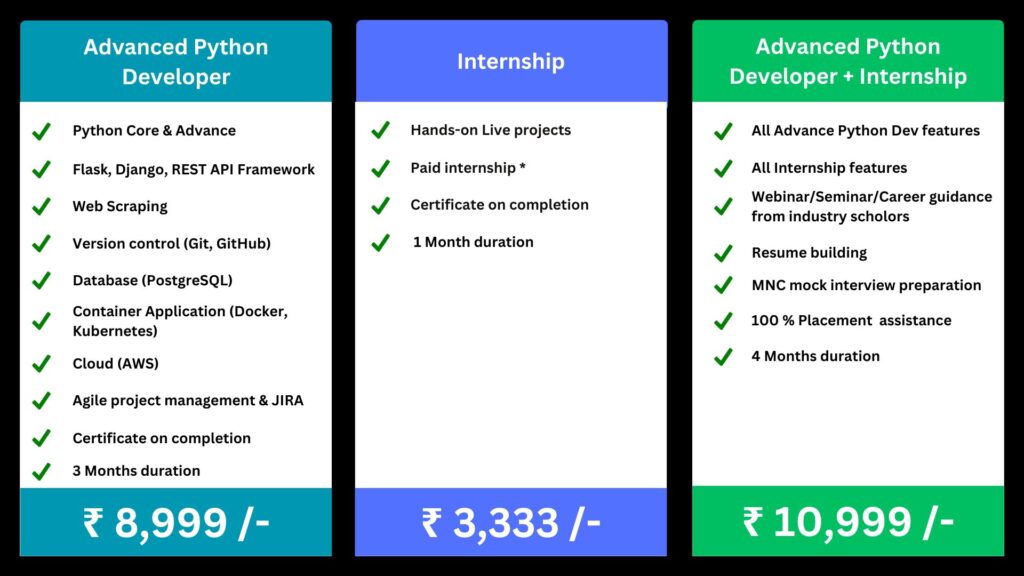
Why Python
- Versatility: Python is a versatile programming language suitable for a wide range of applications, including web development, data analysis, artificial intelligence, machine learning, scientific computing, automation, and more.
- Ease of Learning: Python is renowned for its simple and easy-to-understand syntax, making it an excellent choice for beginners.
- Job Opportunities: Python is one of the most in-demand programming languages in the job market. Proficiency in Python opens up a plethora of job opportunities across various industries, including tech giants, startups, research institutions, finance, healthcare, and academia.
- Data Science and Machine Learning: Python has become the de facto language for data science and machine learning due to its extensive libraries such as NumPy, Pandas, Matplotlib, and scikit-learn.
- Automation and Scripting: Python’s simplicity and versatility make it ideal for automating repetitive tasks, writing scripts, and developing applications quickly.
- High Demand and Future Growth: The demand for Python developers continues to rise steadily, driven by its widespread adoption in various fields.